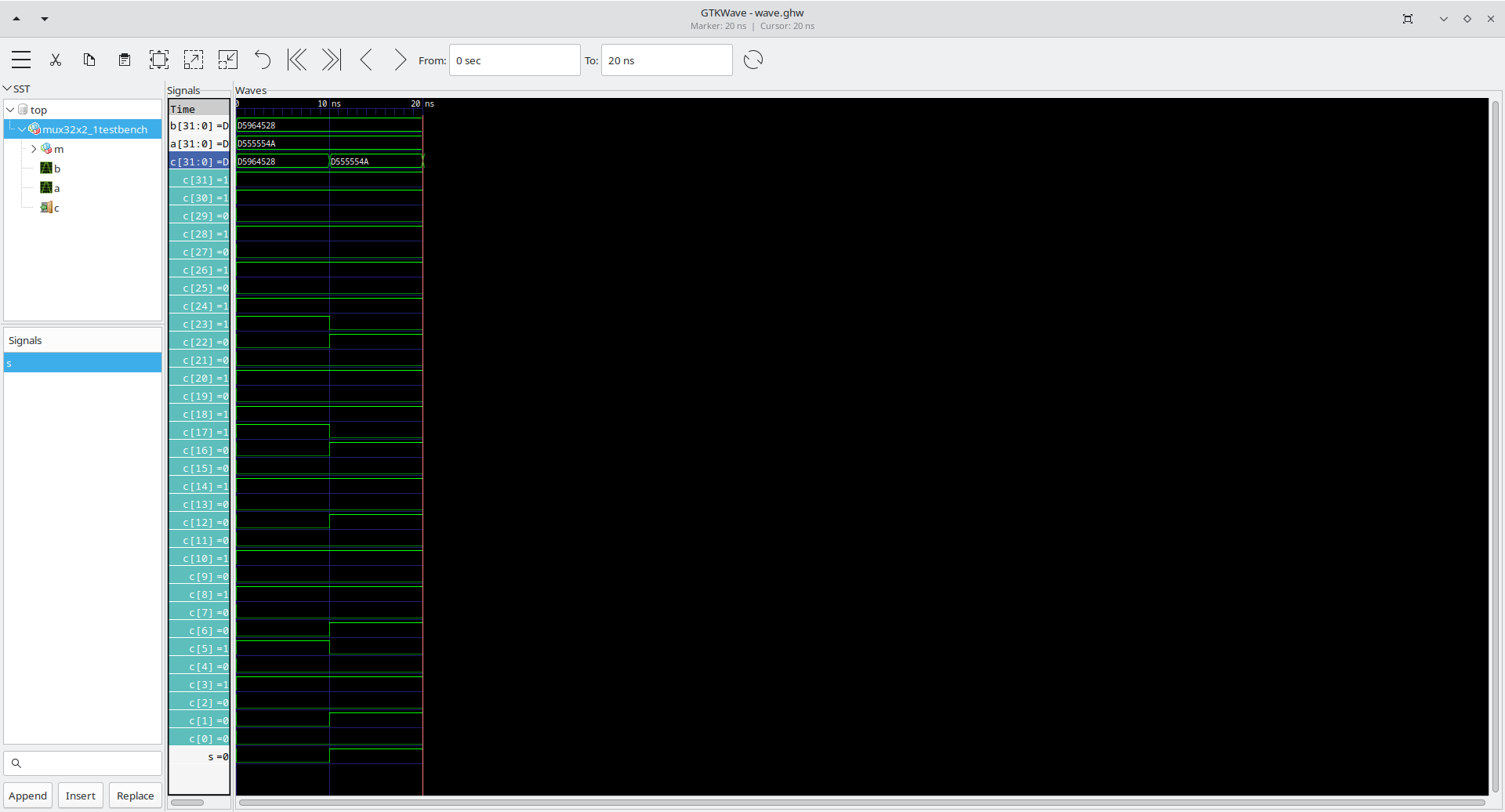
Code: Alles auswählen
library ieee;
use ieee.std_logic_1164.all;
entity rslatch is
port (
r, s: in std_logic;
q: out std_logic
);
end;
architecture verhalten of rslatch is
signal q1, q2: std_logic;
signal init: std_logic;
begin
init <= '0' after 0 ns, '1' after 1 ns;
q1 <= '1' when (init='0') else
(q2 nor r);
q2 <= '0' when (init='0') else
(q1 nor s);
q <= q1;
end;
library ieee;
use ieee.std_logic_1164.all;
entity clockcontrolledrslatch is
port (
r, s: in std_logic;
c: in std_logic;
q: out std_logic
);
end;
architecture behaviour of clockcontrolledrslatch is
component rslatch
port (
r, s: in std_logic;
q: out std_logic
);
end component;
signal r1, s1: std_logic;
begin
rslatch1: rslatch PORT MAP (r=>r1, s=>s1, q=>q);
s1 <= (s and c);
r1 <= (r and c);
end;
library ieee;
use ieee.std_logic_1164.all;
entity dlatch is
port (
d: in std_logic;
c: in std_logic;
q: out std_logic
);
end;
architecture behaviour of dlatch is
component clockcontrolledrslatch
port (
r, s: in std_logic;
c: in std_logic;
q: out std_logic
);
end component;
signal r1, s1: std_logic;
begin
clockcontrolledrslatch1: clockcontrolledrslatch PORT MAP (r=>r1, s=>s1, q=>q, c=>c);
s1 <= not d;
r1 <= d;
end;
library ieee;
use ieee.std_logic_1164.all;
entity dmsflipflop is
port (
d: in std_logic;
c: in std_logic;
q: out std_logic
);
end;
architecture behaviour of dmsflipflop is
component dlatch
port (
d: in std_logic;
c: in std_logic;
q: out std_logic
);
end component;
signal d1, d2: std_logic;
signal c1, c2: std_logic;
signal q1, q2: std_logic;
begin
dlatch1: dlatch PORT MAP (d=>d1, c=>c1, q=>q1);
dlatch2: dlatch PORT MAP (d=>d2, c=>c2, q=>q2);
c1 <= c;
c2 <= not c;
d1 <= d;
d2 <= q1;
q <= q2;
end;
library ieee;
use ieee.std_logic_1164.all;
entity flipfloptestbench is
port (
q: out std_logic
);
end;
architecture behaviour of flipfloptestbench is
component dmsflipflop
port (
d: in std_logic;
c: in std_logic;
q: out std_logic
);
end component;
signal d, c: std_logic;
begin
dmsflipflop1: dmsflipflop PORT MAP (q=>q, d=>d, c=>c);
c <= '0' after 0 ns, '1' after 20 ns, '0' after 40 ns, '1' after 60 ns, '0' after 80 ns, '1' after 100 ns, '0' after 120 ns, '1' after 140 ns,
'0' after 160 ns, '1' after 180 ns, '0' after 200 ns, '1' after 220 ns, '0' after 240 ns,
'1' after 260 ns, '0' after 280 ns, '1' after 300 ns, '0' after 320 ns, '1' after 340 ns;
d <= '0' after 0 ns, '1' after 150 ns, '0' after 250 ns;
end;
library ieee;
use ieee.std_logic_1164.all;
entity reg32 is
port (
we: in std_logic;
q: out std_logic_vector (31 downto 0);
d: in std_logic_vector (31 downto 0)
);
end;
architecture behaviour of reg32 is
component dmsflipflop
port (
d: in std_logic;
c: in std_logic;
q: out std_logic
);
end component;
begin
l1:
for i in 0 to 31 generate
dmsflipflop1: dmsflipflop PORT MAP (q=>q(i), d=>d(i), c=>we);
end generate;
end;
library ieee;
use ieee.std_logic_1164.all;
entity reg32testbench is
port (
q: out std_logic_vector (31 downto 0)
);
end;
architecture behaviour of reg32testbench is
component reg32
port (
d: in std_logic_vector (31 downto 0);
we: in std_logic;
q: out std_logic_vector (31 downto 0)
);
end component;
signal d: std_logic_vector (31 downto 0);
signal we: std_logic;
begin
reg32a: reg32 PORT MAP (d=>d, q=>q, we=>we);
d(0) <= '1';
d(1) <= '0';
d(2) <= '1';
d(3) <= '0';
d(4) <= '1';
d(5) <= '0';
d(6) <= '1';
d(7) <= '0';
d(8) <= '1';
d(9) <= '1';
d(10) <= '0';
d(11) <= '1';
d(12) <= '0';
d(13) <= '1';
d(14) <= '0';
d(15) <= '1';
d(16) <= '0';
d(17) <= '1';
d(18) <= '0';
d(19) <= '1';
d(20) <= '0';
d(21) <= '1';
d(22) <= '0';
d(23) <= '1';
d(24) <= '0';
d(25) <= '1';
d(26) <= '0';
d(27) <= '1';
d(28) <= '0';
d(29) <= '1';
d(30) <= '0';
d(31) <= '1';
we <= '0' after 0 ns, '1' after 10 ns, '0' after 20 ns, '1' after 30 ns, '0' after 40 ns, '1' after 60 ns, '0' after 80 ns;
end;
library ieee;
use ieee.std_logic_1164.all;
entity addressdecoder5to32 is
port (
a: in std_logic_vector (4 downto 0);
b: out std_logic_vector (31 downto 0)
);
end;
architecture behaviour of addressdecoder5to32 is
begin
-- 00000
b (0) <= not a (4) and not a (3) and not a (2) and not a (1) and not a (0);
-- 00001
b (1) <= not a (4) and not a (3) and not a (2) and not a (1) and a (0);
-- 00010
b (2) <= not a (4) and not a (3) and not a (2) and a (1) and not a (0);
-- 00011
b (3) <= not a (4) and not a (3) and not a (2) and a (1) and a (0);
-- 00100
b (4) <= not a (4) and not a (3) and a (2) and not a (1) and not a (0);
-- 00101
b (5) <= not a (4) and not a (3) and a (2) and not a (1) and a (0);
-- 00110
b (6) <= not a (4) and not a (3) and a (2) and a (1) and not a (0);
-- 00111
b (7) <= not a (4) and not a (3) and a (2) and a (1) and a (0);
-- 01000
b (8) <= not a (4) and a (3) and not a (2) and not a (1) and not a (0);
-- 01001
b (9) <= not a (4) and a (3) and not a (2) and not a (1) and a (0);
-- 01010
b (10) <= not a (4) and a (3) and not a (2) and a (1) and not a (0);
-- 01011
b (11) <= not a (4) and a (3) and not a (2) and a (1) and a (0);
-- 01100
b (12) <= not a (4) and a (3) and a (2) and not a (1) and not a (0);
-- 01101
b (13) <= not a (4) and a (3) and a (2) and not a (1) and a (0);
-- 01110
b (14) <= not a (4) and a (3) and a (2) and a (1) and not a (0);
-- 01111
b (15) <= not a (4) and a (3) and a (2) and a (1) and a (0);
-- 10000
b (16) <= a (4) and not a (3) and not a (2) and not a (1) and not a (0);
-- 10001
b (17) <= a (4) and not a (3) and not a (2) and not a (1) and a (0);
-- 10010
b (18) <= a (4) and not a (3) and not a (2) and a (1) and not a (0);
-- 10011
b (19) <= a (4) and not a (3) and not a (2) and a (1) and a (0);
-- 10100
b (20) <= a (4) and not a (3) and a (2) and not a (1) and not a (0);
-- 10101
b (21) <= a (4) and not a (3) and a (2) and not a (1) and a (0);
-- 10110
b (22) <= a (4) and not a (3) and a (2) and a (1) and not a (0);
-- 10111
b (23) <= a (4) and not a (3) and a (2) and a (1) and a (0);
-- 11000
b (24) <= a (4) and a (3) and not a (2) and not a (1) and not a (0);
-- 11001
b (25) <= a (4) and a (3) and not a (2) and not a (1) and a (0);
-- 11010
b (26) <= a (4) and a (3) and not a (2) and a (1) and not a (0);
-- 11011
b (27) <= a (4) and a (3) and not a (2) and a (1) and a (0);
-- 11100
b (28) <= a (4) and a (3) and a (2) and not a (1) and not a (0);
-- 11101
b (29) <= a (4) and a (3) and a (2) and not a (1) and a (0);
-- 11110
b (30) <= a (4) and a (3) and a (2) and a (1) and not a (0);
-- 11111
b (31) <= a (4) and a (3) and a (2) and a (1) and a (0);
end;
library ieee;
use ieee.std_logic_1164.all;
entity addressdecoder5to32testbench is
port (
q: out std_logic_vector (31 downto 0)
);
end;
architecture behaviour of addressdecoder5to32testbench is
component addressdecoder5to32
port (
a: in std_logic_vector (4 downto 0);
b: out std_logic_vector (31 downto 0)
);
end component;
signal d: std_logic_vector (4 downto 0);
begin
addressdecoder5to32a: addressdecoder5to32 PORT MAP (b=>q, a=>d);
d (0) <= '0' after 0 ns, '1' after 10 ns, '0' after 20 ns, '1' after 30 ns, '0' after 40 ns, '1' after 50 ns, '0' after 60 ns, '1' after 70 ns, '0' after 80 ns, '1' after 90 ns, '0' after 100 ns, '1' after 110 ns, '0' after 120 ns, '1' after 130 ns, '0' after 140 ns, '1' after 150 ns, '0' after 160 ns, '1' after 170 ns, '0' after 180 ns, '1' after 190 ns, '0' after 200 ns, '1' after 210 ns, '0' after 220 ns, '1' after 230 ns, '0' after 240 ns, '1' after 250 ns, '0' after 260 ns, '1' after 270 ns, '0' after 280 ns, '1' after 290 ns, '0' after 300 ns, '1' after 310 ns;
d (1) <= '0' after 0 ns, '1' after 20 ns, '0' after 40 ns, '1' after 60 ns, '0' after 80 ns, '1' after 100 ns, '0' after 120 ns, '1' after 140 ns, '0' after 160 ns, '1' after 180 ns, '0' after 200 ns, '1' after 220 ns, '0' after 240 ns, '1' after 260 ns, '0' after 280 ns, '1' after 300 ns, '0' after 320 ns, '1' after 340 ns;
d (2) <= '0' after 0 ns, '1' after 40 ns, '0' after 80 ns, '1' after 120 ns, '0' after 160 ns, '1' after 200 ns, '0' after 240 ns, '1' after 280 ns, '0' after 320 ns, '1' after 360 ns;
d (3) <= '0' after 0 ns, '1' after 80 ns, '0' after 160 ns, '1' after 240 ns, '0' after 320 ns, '1' after 400 ns;
d (4) <= '0' after 0 ns, '1' after 160 ns;
end;
library ieee;
use ieee.std_logic_1164.all;
entity registerset32x32 is
port (
writereg: in std_logic_vector (4 downto 0);
readreg1: in std_logic_vector (4 downto 0);
readreg2: in std_logic_vector (4 downto 0);
readport1: out std_logic_vector (31 downto 0);
readport2: out std_logic_vector (31 downto 0);
writeport: in std_logic_vector (31 downto 0);
we: in std_logic
);
end;
architecture behaviour of registerset32x32 is
component reg32
port (
d: in std_logic_vector (31 downto 0);
we: in std_logic;
q: out std_logic_vector (31 downto 0)
);
end component;
component addressdecoder5to32
port (
a: in std_logic_vector (4 downto 0);
b: out std_logic_vector (31 downto 0)
);
end component;
signal writereg1: std_logic_vector (31 downto 0);
begin
addressdecoder5to32a: addressdecoder5to32 PORT MAP (a=>writereg, b=>writereg1);
l1:
for i in 0 to 31 generate
reg32a: reg32 PORT MAP (we=>writereg1(i),d=>writeport,q=>readport1); -- WARNING - WARNING
end generate;
end;
library ieee;
use ieee.std_logic_1164.all;
entity mux32x2_1 is
port (
b: in std_logic_vector (31 downto 0);
a: in std_logic_vector (31 downto 0);
c: out std_logic_vector (31 downto 0);
s: in std_logic
);
end;
architecture behaviour of mux32x2_1 is
begin
l1:
for i in 0 to 31 generate
c (i) <= (a (i) and s) or (b (i) and not s);
end generate;
end;
library ieee;
use ieee.std_logic_1164.all;
entity mux32x2_1testbench is
port (
c: out std_logic_vector (31 downto 0)
);
end;
architecture behaviour of mux32x2_1testbench is
component mux32x2_1
port (
b: in std_logic_vector (31 downto 0);
a: in std_logic_vector (31 downto 0);
c: out std_logic_vector (31 downto 0);
s: in std_logic
);
end component;
signal a: std_logic_vector (31 downto 0);
signal b: std_logic_vector (31 downto 0);
signal s: std_logic;
begin
m: mux32x2_1 PORT MAP (b=>b, a=>a, c=>c, s=>s);
a (0) <= '0';
a (1) <= '1';
a (2) <= '0';
a (3) <= '1';
a (4) <= '0';
a (31) <= '1';
a (5) <= '0';
a (6) <= '1';
a (7) <= '0';
a (8) <= '1';
a (9) <= '0';
a (10) <= '1';
a (11) <= '0';
a (12) <= '1';
a (13) <= '0';
a (14) <= '1';
a (15) <= '0';
a (16) <= '1';
a (17) <= '0';
a (18) <= '1';
a (19) <= '0';
a (20) <= '1';
a (21) <= '0';
a (22) <= '1';
a (23) <= '0';
a (24) <= '1';
a (25) <= '0';
a (26) <= '1';
a (27) <= '0';
a (28) <= '1';
a (29) <= '0';
a (30) <= '1';
b (0) <= '0';
b (1) <= '0';
b (2) <= '0';
b (3) <= '1';
b (4) <= '0';
b (31) <= '1';
b (5) <= '1';
b (6) <= '0';
b (7) <= '0';
b (8) <= '1';
b (9) <= '0';
b (10) <= '1';
b (11) <= '0';
b (12) <= '0';
b (13) <= '0';
b (14) <= '1';
b (15) <= '0';
b (16) <= '0';
b (17) <= '1';
b (18) <= '1';
b (19) <= '0';
b (20) <= '1';
b (21) <= '0';
b (22) <= '0';
b (23) <= '1';
b (24) <= '1';
b (25) <= '0';
b (26) <= '1';
b (27) <= '0';
b (28) <= '1';
b (29) <= '0';
b (30) <= '1';
s <= '0' after 0 ns, '1' after 10 ns, '0' after 20 ns;
end;